How to Run a Python Script With Arguments in Unreal Engine 4
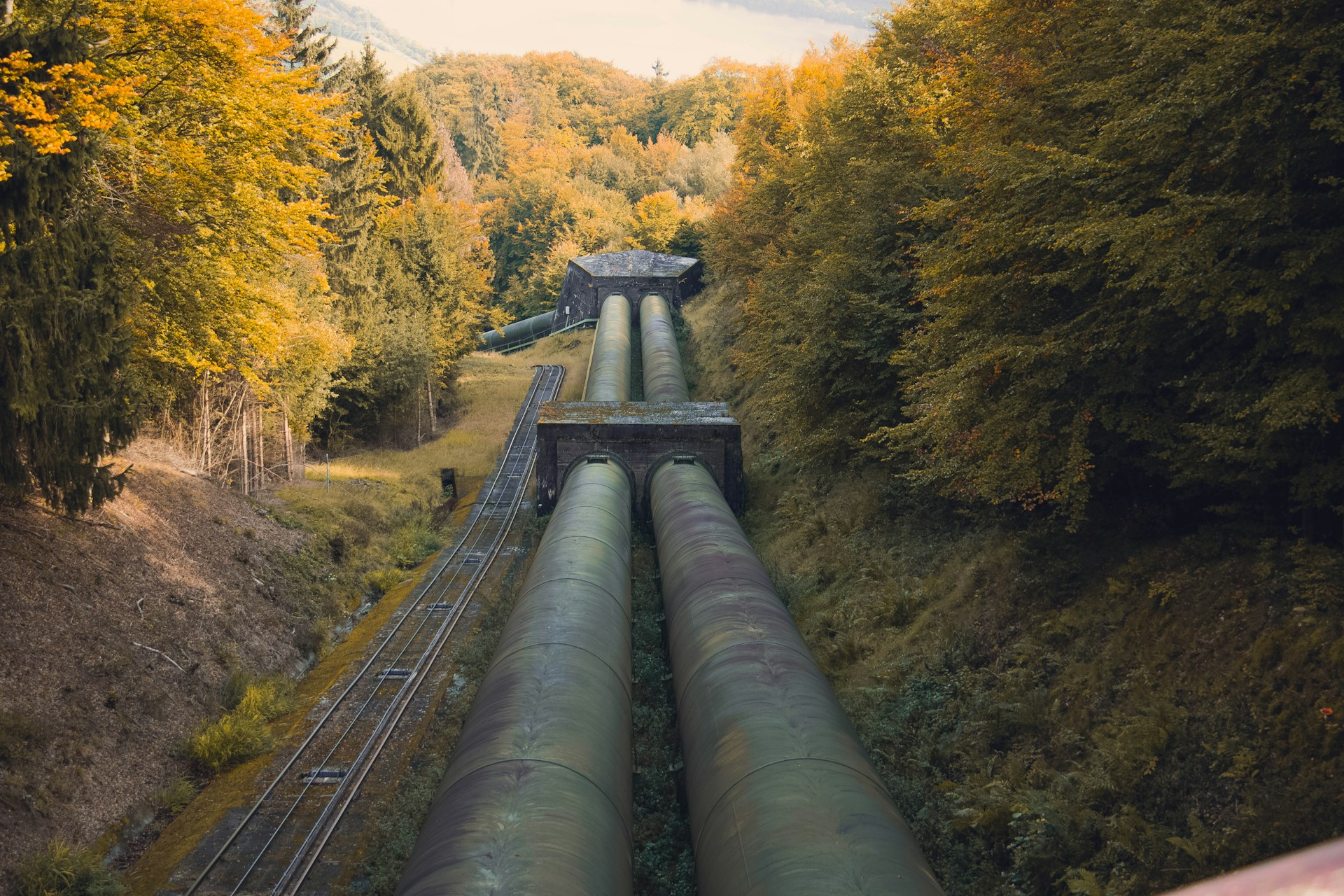
You can run Unreal Engine Python scripts from the commandline to pipeline UE4 with other apps or automate repetitive tasks for your projects.
Building the Command#
While the UE4 documentation lists a few different ways to run Python scripts, the approach I’ll be going over has given me the most consistent results. This approach works great for running scripts for projects offline or even batching work for a project you currently have open. Here’s a template explaining what the command should look like:
<path to UE4Editor-Cmd.exe> <project to operate on> -ExecutePythonScript="<path to script> < optional space-separated python script arguments>"
One detail to note is that the Python script arguments are all part of
the -ExecutePythonScript
argument so I encapsulate them along with the
script path in quotes to pass them in together. Here’s an example of
what the command might look like for an FBX import script:
"C:\Program Files\Epic Games\UE_4.25\Engine\Binaries\Win64\UE4Editor-Cmd.exe" "C:\Path\To\Project.uproject" -ExecutePythonScript="C:\Path\To\do_import.py C:\Path\To\model.fbx /Game/MyProject/Level/Test"
I’m using quotes around the UE4Editor-Cmd.exe
and also the project path
so that I don’t need to worry about escaping the backslashes on Windows.
Note that this will open the UE4Editor, run the script, and close the
UE4Editor automatically. From what I’ve found, there isn’t a reliable
way to run a Python script in headless mode at this time, but you can
still use this approach to run scripts unsupervised.
Accessing the Arguments in Python#
Once you have UE4 calling your script, you can access the arguments the
same way you would when you execute a Python script with a standard
python interpreter. The arguments can be accessed with sys.argv
.
import sys
# This is the script file you're running.
print(sys.argv[0])
## Result: C:\\Path\\To\\do_import.py
# sys.argv[1] and onward gives you access to your scripts arguments.
print(sys.argv[1])
## Result: C:\\Path\\To\\model.fbx
Helpful Flags#
For easier debugging while working on your script, I recommend using
-stdout
and -FullStdOutLogOutput
. It was tricky to find these flags,
but without these, you won’t be able to see your print statements or
Python error output. Here’s an example of the full command put together:
"C:\Program Files\Epic Games\UE_4.25\Engine\Binaries\Win64\UE4Editor-Cmd.exe" "C:\Path\To\Project.uproject" -stdout -FullStdOutLogOutput -ExecutePythonScript="C:\Path\To\do_import.py C:\Path\To\model.fbx /Game/MyProject/Level/Test"
Conclusion#
That’s all you need to get started running UE4 Python scripts from the commandline. For more information about other ways to run Python scripts interactively via the Console or Blueprints, check out the Unreal Engine Python Documentation.