How to Use PyCharm’s Remote Debugging With Maya
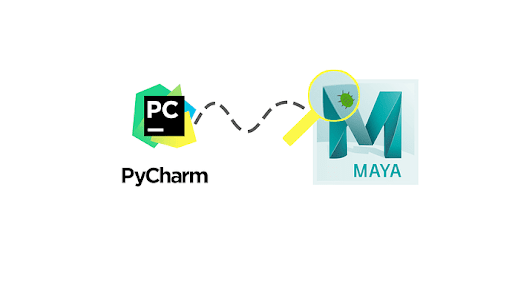
Connecting PyCharm’s debugger to Maya will help you efficiently introspect your code as it runs, find your bugs, and fix them.
I’ll show you what you need to do to connect PyCharm to Maya so that PyCharm can monitor your code as it runs. Before you get started, remote debugging is a feature that is only available with PyCharm Professional. You can trial the full version of PyCharm to give remote debugging a whirl if you’re still on the Community edition.
Creating a Remote Debugging Configuration#
First, you’ll need to configure the remote debugger within PyCharm.
- Click Run –> Edit Configurations…
- Click the plus icon
to add a new configuration.
- Choose Python Debug Server as the template.
- Name your new configuration “Maya Remote Debug”
- Set Port to “9001”
- You can choose a different port number if you know what you’re doing. You’ll need to remember what number you used for the next section.
- Uncheck Suspend after connect
- Click OK
- You should see your new debug configuration selected on the top right of the main window. Select it from that dropdown if it’s not.
Connect Your Executing Code To The Debug Server#
There are a few ways to connect to the debugger, but I have found it most reliable to inject the connecting code somewhere near the entry point of the code I’m testing. Here’s the code that I inject to connect:
import sys
# This should be the path your PyCharm installation
pydevd_egg = r"C:\Program Files\JetBrains\PyCharm 2020.3.3\debug-eggs\pydevd-pycharm.egg"
if not pydevd_egg in sys.path:
sys.path.append(pydevd_egg)
import pydevd
# This clears out any previous connection in case you restarted the debugger from PyCharm
pydevd.stoptrace()
# 9001 matches the port number that I specified in my configuration
pydevd.settrace('localhost', port=9001, stdoutToServer=True, stderrToServer=True, suspend=False)
This is fairly simple. It’s just adding the pydevd egg to my PYTHONPATH so that I’m able to subsequently import that module and call pydevd.settrace to connect. Now, how am I injecting it in my project? If I’m working on my glTF exporter and my test code is something like, glTFExport.export(r"C:\path\to\my\file.gltf"), then I add it at the top of my export function. This way it connects/reconnects whenever I run my test. For example:
def export(file_path=None, resource_format='bin', anim='keyed', vflip=True, selection=False):
import sys
pydevd_egg = r"C:\Program Files\JetBrains\PyCharm 2020.3.3\debug-eggs\pydevd-pycharm.egg"
if not pydevd_egg in sys.path:
sys.path.append(pydevd_egg)
import pydevd
pydevd.stoptrace()
pydevd.settrace('localhost', port=9001, stdoutToServer=True, stderrToServer=True, suspend=False)
GLTFExporter(file_path, resource_format, anim, vflip).run()
Test the Debugger#
- Click to the right of a line number in your code where you want to stop. It’ll add a red dot to indicate that you’ve added a breakpoint.
breakpoint
- You should set the breakpoint at some point after your connection code. That’s why it helps to put the connection code near your entry point.
- Click the debug icon
at the top right of the window to start the debugger.
- You should see a message in the console indicating the debug server has started and “Waiting for process connection…”
- Run your test code in Maya’s script editor.
If all went well, Maya will have stopped responding, but PyCharm will show you your current breakpoint and will be waiting for you to respond.
What If I Don’t Run My Code From My Repository?#
If you’re like me, you might copy your modules to the scripts directory or some other place where Maya can pick it up instead of telling Maya to read directly from the repo you’re working from. If that’s the case, the debugger will still connect and halt at an exception, but your breakpoints won’t work. PyCharm unfortunately won’t automatically relate the breakpoints in your project to the same code that’s running from another location. You could open the copied files in PyCharm and set the breakpoints there, but that’s messy. Fortunately, Path Mapping will help remedy this issue. Let’s update the debug configuration to account for this:
- Click Run –> Edit Configurations…
- Click the folder icon folder icon in the Path mappings field.
- Click the plus icon
in the Edit Path Mappings window to add a new mapping.
- For the Local path, you’ll want to put the path to the code in your PyCharm project
- For the Remote path, you’ll give it the path to where you’re copying your code for Maya to load it.
- Click OK and completely close out the configuration settings.
Now, PyCharm will be able to associate the breakpoints from your project with the executing code from Maya.
Conclusion#
I hope this helps you get started with remote debugging your Maya scripts and plugins. If this is your first time using PyCharm’s debugging tools, I think you’ll find them very useful. They can make it really easy to get to the bottom of even the nastiest of bugs.