How to Use a Git Repository as a Pip Dependency
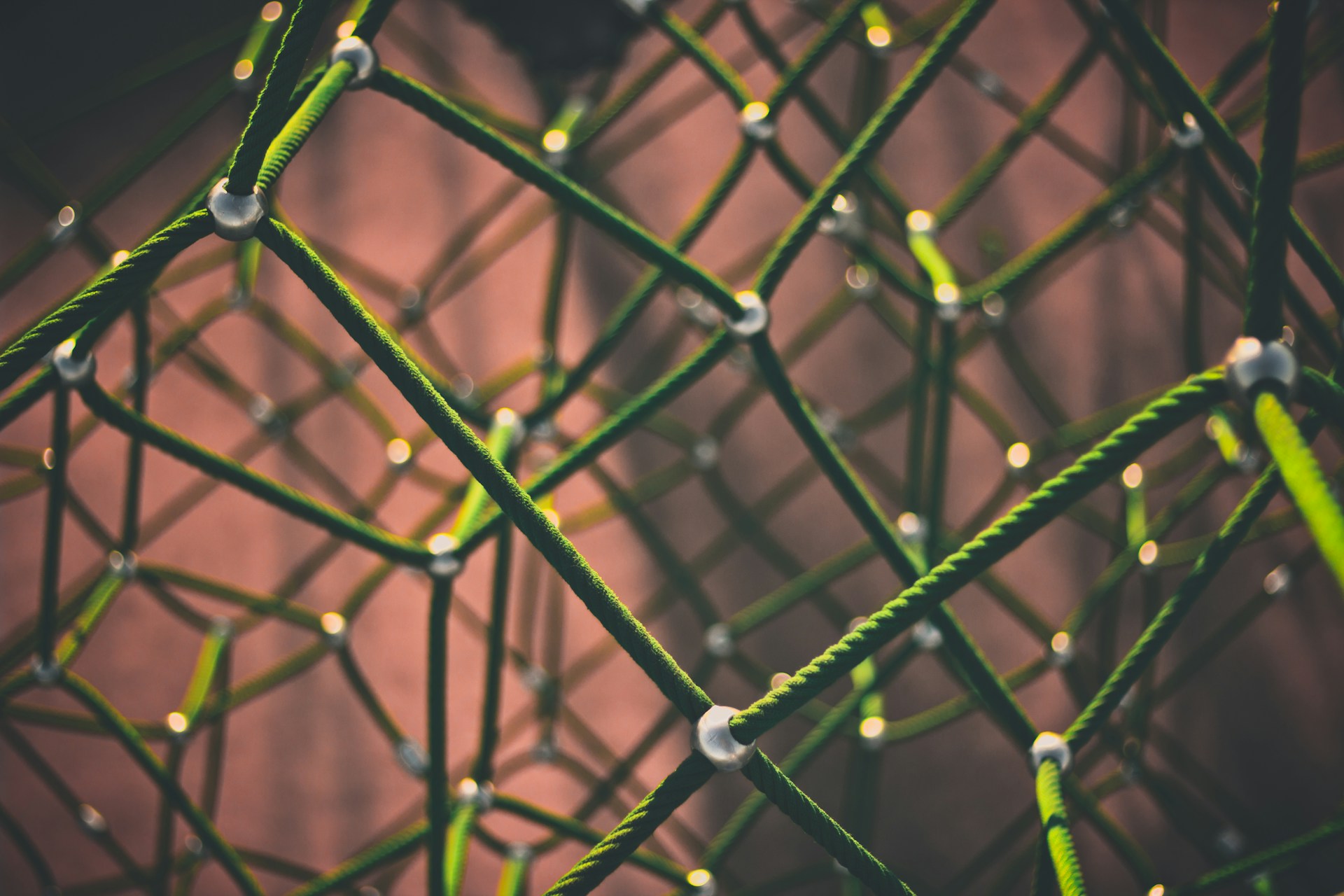
Did you know you can install a python library using a link to a git repository instead of package index?
It can be handy to use pip to install a project dependency directly from a git repository instead of from a Python package index. I’ll show you why you might want to do that as a part of your package management and how to do it.
Why Would You Want to pip install
From a Git Repository?#
- You may want to quickly test out some code from a colleague.
- You may have a library that you want to use in multiple projects, but it doesn’t warrant creating a python package.
- You made a fix to a third party project and can’t wait for the pull request to be merged and deployed. You can use your fork and then switch to an official release later.
How to pip install
From a Git Repository#
If this is a new repo, you’ll minimally need a setup.py so that pip can carry out the install. Other than that, it really just boils down to giving pip a git repository URL.
# You'll want to do something in the form of
pip install git+<repository_url>
# For example
pip install git+https://github.com/matiascodesal/git-for-pip-example.git
# Check that it worked
python -c "import greetings;greetings.hello()"
You can explicitly call out the package name that you’re installing with
#egg=
# The syntax
pip install git+<repository_url>#egg=<package_name>
# Explicit package name example
pip install git+https://github.com/matiascodesal/git-for-pip-example.git#egg=git-for-pip-example
There are also a number of different ways to specify a version of the repository that you want to fetch. It’s wise to use one of these methods to lock your dependency so that you get consistent results.
# Use a commit SHA
pip install git+https://github.com/matiascodesal/git-for-pip-example.git@4045597#egg=git-for-pip-example
# Use a tag
pip install git+https://github.com/matiascodesal/[email protected]#egg=git-for-pip-example
# Use a branch called "GreetingArg"
pip install git+https://github.com/matiascodesal/git-for-pip-example.git@GreetingArg#egg=git-for-pip-example
How to Include the Dependency in a requirements.txt
#
If a git repository dependency is going to live in a project for more
than just testing, you’ll likely want to add it to your
requirements.txt. There’s a bug with pip freeze
where the git
repository dependency wasn’t being output by pip freeze
. That was only
recently fixed in pip 20.1. I’ll show you the workaround for older
versions of pip and the new way to list the dependency. The workaround
for older versions of pip is to use the -e
or --editable
flag:
# The pip command with "-e"
pip install -e git+https://github.com/matiascodesal/[email protected].0#egg=my-git-package
# What that should look like in your requirements.txt
packageA==1.2.3
-e https://github.com/matiascodesal/[email protected].0#egg=my-git-package
packageB==4.5.6
For pip 20.1 or newer, you no longer need the -e
flag:
# Just put the pip install argument straight into your requirements.txt
packageA==1.2.3
git+https://github.com/matiascodesal/[email protected].0#egg=my-git-package
packageB==4.5.6
# Or you can use the preferred PEP 440 direct URL syntax
packageA==1.2.3
git-for-pip-example @ git+https://github.com/matiascodesal/[email protected]
packageB==4.5.6
I hope this gives you some flexibility in managing your projects while still being mindful of tracking your dependencies and deploying code in a consistent and reliable manner.