Spice Up Your Qt for Python With Font Awesome Icons
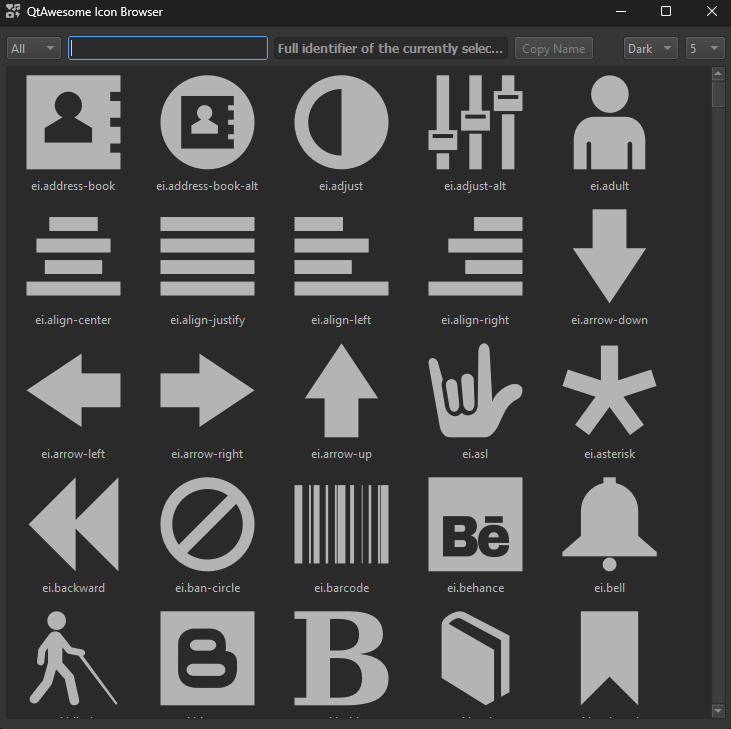
Elevate your UI design by adding Font Awesome icons to your PyQt5 or PySide2 project. QtAwesome makes it really easy.
With the QtAwesome package, you can be up and running with thousands of icons at your disposal in a matter of minutes. Start out by installing the QtAwesome package:
pip install qtawesome
qta-browser
Using QtAwesome is simple and it’ll work with with PyQt or PySide out of the box. Here’s how to create a button with an icon:
from PySide2.QtWidgets import QApplication, QPushButton, QWidget, QVBoxLayout
# The only import you need for icons
import qtawesome as qta
app = QApplication()
window = QWidget()
layout = QVBoxLayout()
# icon name from the qta-browser
icon = qta.icon("fa5s.home")
button = QPushButton(icon, "Home")
# alternatively add an icon to an
# existing widget with: widget.setIcon(icon)
layout.addWidget(button)
window.setLayout(layout)
window.show()
app.exec_()
Want to set a color for the icon? The API can handle that for you:
...
# set a color with QColor or a hex string
icon = qta.icon("ei.bell", color="#fd0")
button = QPushButton(icon, "Bell")
layout.addWidget(button)
window.setLayout(layout)
window.show()
app.exec_()
The QtAwesome documentation has some more examples for stacking icons, adding animation, adding transformations, and automatically changing color or icon type based on different widget states. Hope that helps you get started towards adding some polish to your user interfaces.